I came across a pretty interesting series of articles when I was reading through Eric Lippert's MSDN blog today. Here are some of the things i grasped from him.
What is the first thing that comes to your mind when someone asks from you "What is the difference between value types and reference types?" Well I bet you will reply saying that value types are allocated in the stack and reference types are allocated in heap, right! Well you are correct that's what the C# documentation tells us. Then why don't we just call them stack types and heap types instead of value and reference. Well that's because that is not true most of the time.
Let's take the following class as an example.
public class Demo
{
private int ValueOne = 10;
public int GetValue()
{
return ValueOne;
}
}
Here the variable "ValueOne" is defined inside the "Demo" class. Here although "ValueOne" is a value type integer variable it is an integral part of the "Demo" s instance memory. So it is allocated inside the heap.
Let's have a look at how this happens.
Before we dwell in to it, let's see what does the terms "short lived" and "long lived" stands for in context of variables
When a method is called an activation period is defined for that method, which can be defined as the time between the start of the method execution and its successful return or a throwing of an exception. Code in the method may require allocation of storage in memory for its variables. Let's look at a simple example
public int GetSum()
{
int x = 10;
int y = 20;
int sum = Add(x, y);
return sum;
}
public int Add(int i, int j)
{
return i + j;
}
Here when the method GetSum calls method Add, the use of the storage locations for the parameters passed to Add and the value returned by Add is required by GetSum. This means that the required life time of the storage location is actually longer than the activation period of the method, thus making it a long lived variable or storage.
So what does long/short lived stands for "If the required life time of a variable/storage is longer than its current method execution's activation period that variable is classified as a long lived variable otherwise a short lived one"
So how can value type variables get allocated inside the heap? Let's get to it one step at a time
What happens is that during the compile time analysis if a local or a temporary variable is found to be un-used after the methods activation time it is stored on stack or register otherwise on heap. Depending on this model we can see that references and instances of value types are the same as far as their storage is concerned, but they can go on to either of those above mentioned storage locations depending on their life time.
So that leaves us with an explanation that actually does not explain any concrete thing.
Then what is the main, clearly distinctive difference between them. The best explanation is that "Value types are always copied by value and Reference types are always copied by reference" and we can also go on to say that "Value types semantically represents a value and Reference types are a semantic reference to something".
Welcome
"Happy are those who dream dreams and are ready to pay the price to make them come true"
About Me
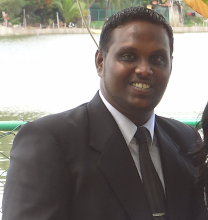
My Home @ google Map
- Dhanushka Athukorala
- Colombo, Western Province, Sri Lanka
- I'm a Microsoft Certified Professional (MCP-ASP.net) who is currently working as a Software Engineer @ Aeturnum. I have experience in windows, web, mobile application development using .net framework 2.0, 3.5, 4.0 and .net compact framework 2.0 and 3.5 and i have also carried out development projects in C++, C, VB.net, Java, PHP, Pl/SQL, SQL, XNA, AJAX, Jquery etc...
Followers
Posted by
Dhanushka Athukorala
Monday, December 27, 2010
Subscribe to:
Post Comments (Atom)