I came across an interesting problem when using java script "setSttribute" method with IE. Apparently it does not work with IE versions 5.5 to 7 when we try to use it to set a style to an element.
For all the browsers including IE8 and above this code will work fine when we a want to set a a style attribute to an element using java script.
var elemntToTest=document.createElement('a');
elemntToTest.setAttribute('href', '#');
elemntToTest.setAttribute('style', 'padding:3px');
but in IE 5.5-7 you will need to set the style like this.
elemntToTest.style.cssText = "padding:3px;";
Now it will work fine in all the browsers.
Hope this work around helps!!!
Welcome
About Me
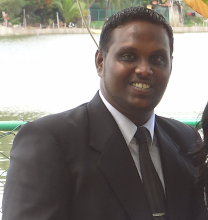
My Home @ google Map
- Dhanushka Athukorala
- Colombo, Western Province, Sri Lanka
- I'm a Microsoft Certified Professional (MCP-ASP.net) who is currently working as a Software Engineer @ Aeturnum. I have experience in windows, web, mobile application development using .net framework 2.0, 3.5, 4.0 and .net compact framework 2.0 and 3.5 and i have also carried out development projects in C++, C, VB.net, Java, PHP, Pl/SQL, SQL, XNA, AJAX, Jquery etc...
Blog Archive
-
▼
2010
(16)
-
▼
November
(8)
- JavaScript setAttribute not working for IE
- Flot, A pure JQuery Charting tool and How to get t...
- Object Oriented JQuery/JavaScript
- Store a value in cookie from a class project in AS...
- Truncate a paragraph to the last word
- Remove duplicate values from a list
- Set the check status of all check boxes in a page ...
- Get all the selected check box IDs in page using J...
-
▼
November
(8)
Followers
As we all know there are some pretty amazing and powerful charting tools available for web developers to use, some are commercial and some are free. But a main disadvantage i saw in many of them is that, almost all of them use flash to draw graphics and manipulate settings.
But there is some pretty sleek free JQuery chart plug-ins available out there. To name a few Flot, Google Charts, jQuery Chart, jQchart, TufteGraph etc...
After a brief R&D Flot attracted me most because of its rich options set and clean, easy to use and maintainable code.
Now I will explain how to draw a simple line chart using Flot in an ASP.net web site.
I will use AJAX calls to fetch data from the server to populate chart data sets in the following example. But rather than using page methods or update panels, I will use simple JQuery AJAX post backs ($.ajax) to accomplish data retrieving tasks.
So here we go...
Let’s take a look at how Flot accepts data to draw its chart. Here is a simple JSON object which will be used to draw the chart line.
{
label: 'Europe (EU27)',
data: [[1999, 3.0], [2000, 3.9]]
}
So let’s create a class similar to this JSON object so we can serialize an object of that class to something similar.
public class Model
{
public string label;
public List<List<int>> data;
}
And let’s write a Helper class containing simple methods that can be used to serialize the Model object to a JSON object.
public static class Helper
{
private static string ToJson(this object obj)
{
var serializer = new DataContractJsonSerializer(obj.GetType());
var ms = new MemoryStream();
serializer.WriteObject(ms, obj);
var json = Encoding.Default.GetString(ms.ToArray());
return json;
}
public static string GetJason(Model dataModel)
{
return dataModel.ToJson();
}
}
* DataContractJsonSerializer is inside the System.Runtime.Serialization.Json namespace
Now we will write a simple web method (web service method) that can be called from the client side JavaScript.
[WebMethod]
public static string GetData()
{
var dataModel = new Model {label = "Sample Chart"};
var dataList = new List<List<int>>();
for (int i = 1; i <>
{
var data = new List
dataList.Add(data);
}
dataModel.data = dataList;
return Helper.GetJason(dataModel);
}
This function will return a serialized data object like this.
{
"data":[[1,9],[2,12],[3,17],[4,24],[5,33],[6,44],[7,57],[8,72],[9,89]],
"label":"Sample Chart"
}
Now that's all from the server side. Let’s look at the client side javascript functions
In the aspx page add references to jquery.js and jquery.flot.js (download them from http://code.google.com/p/flot/downloads/list)
And inside the body add a div with the id "placeholder" to hold the chart.
add a button with the id “btnGetData” so we can fetch data upon user request
Here is the sample code to draw the chart using our predefined methods in the server side.
$(document).ready(function() {
//options that can be enabled in the chart, more options are available
var options = {
lines: { show: true },
points: { show: true },
xaxis: { tickDecimals: 0, tickSize: 1 }
};
var data = []; //data used to draw the chart
var placeholder = $("#placeholder"); //chart wrapper
$.plot(placeholder, data, options); //draw the chart
var alreadyFetched = {};
$("#btnGetData").live("click", function(e) {
var button = $(this); //get the clicked button
//execute this function after data is successfully retrieved from the ajax call
function onDataReceived(series) {
series = $.parseJSON(series.d); //get the json object (use the latest jquery script)
var firstcoordinate = '(' + series.data[0][0] + ', ' + series.data[0][1] + ')';
button.siblings('span').text('Fetched ' + series.dabel + ', first point: ' + firstcoordinate);
if (!alreadyFetched[series.label]) {
alreadyFetched[series.label] = true;
data.push(series); //populate data
}
$.plot(placeholder, data, options); //draw the chart
}
var dataUrl = "Default.aspx/GetData"; //server method to get data
$.ajax({
type: "POST",
url: dataUrl,
data: "{}",
contentType: "application/json; charset=utf-8",
dataType: "json",
success: onDataReceived
});
});
});
This will draw a simple line chart on your screen.
Happy charting!!!
All of us are familiar with OOP concepts, right!!
But how many of you have tried to use them in JavaScript.
Here is a simple code example to get you up and running.
First I will create a simple JavaScript class. When uing C# or Java etc... , We use the keyword "class".
To denote a class in JavaScript we use "function".
Here we go...
function Helper() {
$.fn.getCurrentIds = function (name) {
var selectedIds = new Array();
$('input:checkbox[name=' + name + ']:checked').each(function () {
selectedIds.push($(this).val());
});
var idString = "";
for (var i = 0; i < selectedIds.length; i++) {
if (i == selectedIds.length - 1)
idString += selectedIds[i];
else
idString += selectedIds[i] + ',';
}
return idString;
};
this.setAllSelectedIds = function (checkBoxName, hdnId) {
var hdnControlString = '#' + hdnId + '';
if ($(hdnControlString)) {
if ((!($(hdnControlString).val())) || $(hdnControlString).val() == "")
$(hdnControlString).val($.fn.getCurrentIds(checkBoxName));
else
if ($.fn.getCurrentIds(checkBoxName) != "")
$(hdnControlString).val($(hdnControlString).val() + ',' + $.fn.getCurrentIds(checkBoxName));
}
};
this.setCheckboxStatus = function (hdnId) {
var hdnControlString = '#' + hdnId + '';
if ($(hdnControlString) && $(hdnControlString).val()) {
if ($(hdnControlString).val() != "") {
var idArray = $(hdnControlString).val().split(',');
$.each(idArray, function (index, value) {
if ("input:checkbox[value=" + value + "]")
$("input:checkbox[value=" + value + "]").attr("checked", true);
});
}
}
};
this.getAllSelectedIds = function (hdnId) {
var hdnControlString = '#' + hdnId + '';
var idString = "";
if ($(hdnControlString))
idString = $(hdnControlString).val();
if (!idString)
idString = "";
return idString;
};
}
Here I have defined some useful common utility functions which will be used in and out side of the class.
if you look closely, you will see that the function "getCurrentIds" is called from another method or a function within the class that is "setAllSelectedIds" we have used JQuery pointers to do so - "$.fn.getCurrentIds(checkBoxName)"
what about out side of the class.
Its the same as any OOP based language.
-Create an object of the class
-Call object methods
here is an example
var helperObj = new Helper();
helperObj.setAllSelectedIds(itemCheckBoxName, hdnAllChkIds);//returns void
var allItemsSelected = helperObj.getAllSelectedIds(hdnAllChkIds);//returns a string containing all the selected check box ids.
you can elaborate further on this concept and can use it to write more complex but clean and maintainable code
Hope it helped!!!
You can use the following method to store a string value in cookie using C#.net 4.0
public static void StoreCookie(string name, string valueToStore)
{
var cookie = new HttpCookie(name) {Value = valueToStore, Expires = DateTime.Now.AddYears(1)};
HttpContext.Current.Response.Cookies.Add(cookie);
}
Here classes like "HttpCookie" and "HttpContext" needs to be used in order to access cookies from a class library.
This kind of truncation is always needed if you are displaying a truncated version of a meaningful string in the UI. Rather than simply truncating the list to a specific maximum length it is always handy to chop it to the last meaningful word.
The following lines of code accomplish that task
public static string TruncateToLastWord(string value, int maxLength)
{
string stringToTruncate = value.Substring(0, maxLength);
int indexOfLastSpace = stringToTruncate.LastIndexOf(' ');
string truncatedString = stringToTruncate.Substring(0, indexOfLastSpace);
return string.Format("{0}...", truncatedString);
}
Lets say you have two lists that contains a set of Ids, and some ids are repeated in both the lists.
Here is a simple piece of code to get a concatenated list without the duplicate ids
public static List
{
foreach (var item in newSet)
oldSet.RemoveAll(x=>x==item);
return oldSet;
}
The requirement I am gonna illustrate now comes up in almost all development efforts where a grid or a tabular view is displayed. there is a single check box in the table header where upon click you need to change the checked status of all the check boxes in the grid
Here is a simple bit of code you can use to achieve that task
function(){
$('#' + allCheckBoxName + '').live("change", function (e) {
$("INPUT[type='checkbox']").attr('checked', $('#' + allCheckBoxName + '').is(':checked'));
});
}
Here "allCheckBoxName" is the master check box which is at the top of the grid.
But an issue arise when using this script with a drop down in the same page in IE browsers. that is in IE you need to bind check boxes events first before any other event bindings in order to work them properly. and strangely this is true with drop downs as well. so you face a strange dillema. "How can I bind events for two different kinds of controls at once?"
To overcome this just add the following code segment to the code
if ($.browser.msie) {
$('#' + allCheckBoxName + '').unbind("click");
$('#' + allCheckBoxName + '').live("click", function (e) {
$(this).trigger("change");
});
what it does is it rebinds the check box events after the drop down bind and trigger the change event.
so the completed function will look some thing like this
function () {
if ($.browser.msie) {
$('#' + allCheckBoxName + '').unbind("click");
$('#' + allCheckBoxName + '').live("click", function (e) {
$(this).trigger("change");
});
if (allCheckBoxVisibility) {
$('#' + allCheckBoxName + '').live("change", function (e) {
$("INPUT[type='checkbox']").attr('checked', $('#' + allCheckBoxName + '').is(':checked'));
});
}
}
$('#' + allCheckBoxName + '').live("click", function (e) {
$("INPUT[type='checkbox']").attr('checked', $('#' + allCheckBoxName + '').is(':checked'));
});
}
Hope it helps!!!
lots of guys have asked from me how to do this using java script. here is a small code snippet to help them out
function (name) {
var selectedIds = new Array();
$('input:checkbox[name=' + name + ']:checked').each(function () {
selectedIds.push($(this).val());
});
var idString = "";
for (var i = 0; i < selectedIds.length; i++) {
if (i == selectedIds.length - 1)
idString += selectedIds[i];
else {
idString += selectedIds[i] + ',';
}
}
return idString;
}
Cheers!!!