All of us are familiar with OOP concepts, right!!
But how many of you have tried to use them in JavaScript.
Here is a simple code example to get you up and running.
First I will create a simple JavaScript class. When uing C# or Java etc... , We use the keyword "class".
To denote a class in JavaScript we use "function".
Here we go...
function Helper() {
$.fn.getCurrentIds = function (name) {
var selectedIds = new Array();
$('input:checkbox[name=' + name + ']:checked').each(function () {
selectedIds.push($(this).val());
});
var idString = "";
for (var i = 0; i < selectedIds.length; i++) {
if (i == selectedIds.length - 1)
idString += selectedIds[i];
else
idString += selectedIds[i] + ',';
}
return idString;
};
this.setAllSelectedIds = function (checkBoxName, hdnId) {
var hdnControlString = '#' + hdnId + '';
if ($(hdnControlString)) {
if ((!($(hdnControlString).val())) || $(hdnControlString).val() == "")
$(hdnControlString).val($.fn.getCurrentIds(checkBoxName));
else
if ($.fn.getCurrentIds(checkBoxName) != "")
$(hdnControlString).val($(hdnControlString).val() + ',' + $.fn.getCurrentIds(checkBoxName));
}
};
this.setCheckboxStatus = function (hdnId) {
var hdnControlString = '#' + hdnId + '';
if ($(hdnControlString) && $(hdnControlString).val()) {
if ($(hdnControlString).val() != "") {
var idArray = $(hdnControlString).val().split(',');
$.each(idArray, function (index, value) {
if ("input:checkbox[value=" + value + "]")
$("input:checkbox[value=" + value + "]").attr("checked", true);
});
}
}
};
this.getAllSelectedIds = function (hdnId) {
var hdnControlString = '#' + hdnId + '';
var idString = "";
if ($(hdnControlString))
idString = $(hdnControlString).val();
if (!idString)
idString = "";
return idString;
};
}
Here I have defined some useful common utility functions which will be used in and out side of the class.
if you look closely, you will see that the function "getCurrentIds" is called from another method or a function within the class that is "setAllSelectedIds" we have used JQuery pointers to do so - "$.fn.getCurrentIds(checkBoxName)"
what about out side of the class.
Its the same as any OOP based language.
-Create an object of the class
-Call object methods
here is an example
var helperObj = new Helper();
helperObj.setAllSelectedIds(itemCheckBoxName, hdnAllChkIds);//returns void
var allItemsSelected = helperObj.getAllSelectedIds(hdnAllChkIds);//returns a string containing all the selected check box ids.
you can elaborate further on this concept and can use it to write more complex but clean and maintainable code
Hope it helped!!!
Welcome
"Happy are those who dream dreams and are ready to pay the price to make them come true"
About Me
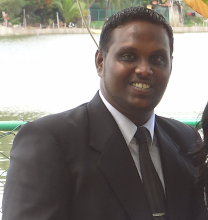
My Home @ google Map
- Dhanushka Athukorala
- Colombo, Western Province, Sri Lanka
- I'm a Microsoft Certified Professional (MCP-ASP.net) who is currently working as a Software Engineer @ Aeturnum. I have experience in windows, web, mobile application development using .net framework 2.0, 3.5, 4.0 and .net compact framework 2.0 and 3.5 and i have also carried out development projects in C++, C, VB.net, Java, PHP, Pl/SQL, SQL, XNA, AJAX, Jquery etc...
Blog Archive
-
▼
2010
(16)
-
▼
November
(8)
- JavaScript setAttribute not working for IE
- Flot, A pure JQuery Charting tool and How to get t...
- Object Oriented JQuery/JavaScript
- Store a value in cookie from a class project in AS...
- Truncate a paragraph to the last word
- Remove duplicate values from a list
- Set the check status of all check boxes in a page ...
- Get all the selected check box IDs in page using J...
-
▼
November
(8)
Followers
Posted by
Dhanushka Athukorala
Tuesday, November 16, 2010
Subscribe to:
Post Comments (Atom)