I came across a pretty interesting series of articles when I was reading through Eric Lippert's MSDN blog today. Here are some of the things i grasped from him.
What is the first thing that comes to your mind when someone asks from you "What is the difference between value types and reference types?" Well I bet you will reply saying that value types are allocated in the stack and reference types are allocated in heap, right! Well you are correct that's what the C# documentation tells us. Then why don't we just call them stack types and heap types instead of value and reference. Well that's because that is not true most of the time.
Let's take the following class as an example.
public class Demo
{
private int ValueOne = 10;
public int GetValue()
{
return ValueOne;
}
}
Here the variable "ValueOne" is defined inside the "Demo" class. Here although "ValueOne" is a value type integer variable it is an integral part of the "Demo" s instance memory. So it is allocated inside the heap.
Let's have a look at how this happens.
Before we dwell in to it, let's see what does the terms "short lived" and "long lived" stands for in context of variables
When a method is called an activation period is defined for that method, which can be defined as the time between the start of the method execution and its successful return or a throwing of an exception. Code in the method may require allocation of storage in memory for its variables. Let's look at a simple example
public int GetSum()
{
int x = 10;
int y = 20;
int sum = Add(x, y);
return sum;
}
public int Add(int i, int j)
{
return i + j;
}
Here when the method GetSum calls method Add, the use of the storage locations for the parameters passed to Add and the value returned by Add is required by GetSum. This means that the required life time of the storage location is actually longer than the activation period of the method, thus making it a long lived variable or storage.
So what does long/short lived stands for "If the required life time of a variable/storage is longer than its current method execution's activation period that variable is classified as a long lived variable otherwise a short lived one"
So how can value type variables get allocated inside the heap? Let's get to it one step at a time
What happens is that during the compile time analysis if a local or a temporary variable is found to be un-used after the methods activation time it is stored on stack or register otherwise on heap. Depending on this model we can see that references and instances of value types are the same as far as their storage is concerned, but they can go on to either of those above mentioned storage locations depending on their life time.
So that leaves us with an explanation that actually does not explain any concrete thing.
Then what is the main, clearly distinctive difference between them. The best explanation is that "Value types are always copied by value and Reference types are always copied by reference" and we can also go on to say that "Value types semantically represents a value and Reference types are a semantic reference to something".
Welcome
About Me
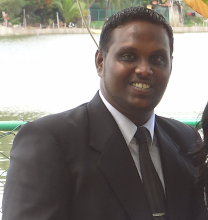
My Home @ google Map
- Dhanushka Athukorala
- Colombo, Western Province, Sri Lanka
- I'm a Microsoft Certified Professional (MCP-ASP.net) who is currently working as a Software Engineer @ Aeturnum. I have experience in windows, web, mobile application development using .net framework 2.0, 3.5, 4.0 and .net compact framework 2.0 and 3.5 and i have also carried out development projects in C++, C, VB.net, Java, PHP, Pl/SQL, SQL, XNA, AJAX, Jquery etc...
Followers
Yesterday I came across an interesting situation. There are some times when you need to break a string in to two parts depending on their character type.
Lets say you have a string like "Welfare Fund - 1000.00" and you need to break the string part and the number part in to two different strings. Well the first thing that comes to mind is iterate the string till you get a number and break it to apart upon hitting on a number type character, right!!
Well then what about a string like "Welfare Fund : 2009 - 3000.00"
Here as you can see, there are numbers in the string part as well.
The solution is simple, all you have to do is iterate through the string by starting from its end, till you reach a blank space. Here is the a method to accomplish that task
public static List<string> BreakString(string stringToBreak)
{
var charArr = stringToBreak.ToCharArray();
var stringAppender = new StringBuilder();
var intAppender = new StringBuilder();
var isSeparated = false;
for (int i = charArr.Length - 1; i > -1; i--)
{
if (isSeparated)
stringAppender.Append(charArr[i]);
else
{
isSeparated = charArr[i] == ' ';
if (!isSeparated)
intAppender.Append(charArr[i]);
}
}
var returnValues = new List<string> { ReverseString(stringAppender.ToString()), ReverseString(intAppender.ToString()) };
return returnValues;
}
public static string ReverseString(string s)
{
var arr = s.ToCharArray();
Array.Reverse(arr);
return new string(arr);
}
You can call it as follows
var input = "Welfare Fund - 1000.00";
var result = BreakString(input);
There are some pretty good Mock object frameworks available out there for .net like NMock, EasyMock, TypeMock, etc... But I think every one will ultimately wind up using Rhino Mock at the end because of its easy to grasp rich feature set. So lets see how we can use Rhino to to mock a layered architecture for unit testing purposes.
I will try to mock the repository and the service layer in the following code in an easy to understand manner
Here are my sample repository and service interfaces (I have used NHibernate and Windsor Castle in this project as the ORM solutionl)
Repository Interface
public interface IInventoryRepository : IRepository
{
List<RecordItem> Get(List<int> ids);
List<RecordItem> Get(List<int> ids, string orderByColumn, string orderType);
}
Service Interface
public interface IInventoryService
{
List<ServiceItem> Get(List<int> ids, int truncLength);
List<ServiceItem> Get(List<int> ids, string orderByColumn, string orderType, int truncLength);
}
As I have described in my previous post lest use Nbuilder to mock the test objects that needs to be returned when the methods in the repository are called
Mock return for List<RecordItem> Get(List<int> ids)
private static List<RecordItem> CreateRecordItems()
{
var items = Builder<RecordItem>.CreateListOfSize(10).WhereAll().Have(x => x.Title = "sample title").WhereAll().Have(x => x.AuthorName = "Dhanushka").Build().ToList();
for (int i = 1; i < items.Count + 1; i++)
{
EntityIdSetter.SetIdOf(items[i - 1], i);
}
return items;
}
Mock return for List<RecordItem> Get(List<int> ids, string orderByColumn, string orderType)
private static List<RecordItem> CreateRecordItemsDesc()
{
var items = Builder<RecordItem>.CreateListOfSize(10).WhereAll().Have(x => x.Title = "sample title").WhereAll().Have(x => x.AuthorName = "Dhanushka").Build().ToList();
var j = items.Count;
for (int i = 1; i < items.Count + 1; i++)
{
EntityIdSetter.SetIdOf(items[i - 1], j);
j--;
}
return items;
}
Now lets use Rhino Mock to mock the Method Calls to the repository
public static IInventoryRepository CreateMockInventoryRepository()
{
var mocks = new MockRepository();
//specify the repository to mock
var mockedRepository = mocks.StrictMock<IInventoryRepository>();
//specify what to return when a specific method is called in the mocked repository
Expect.Call(mockedRepository.Stub(x => x.Get(Arg<List<int>>.Is.Anything)).Return(CreateRecordItems()));
Expect.Call(mockedRepository.Stub(x => x.Get(Arg<List<int>>.Is.Anything, Arg<string>.Is.Anything, Arg<string>.Is.Equal("asc"))).Return(CreateRecordItems()));
Expect.Call(mockedRepository.Stub(x =>x.Get(Arg<List<int>>.Is.Anything, Arg<string>.Is.Anything, Arg<string>.Is.Equal("desc"))).Return(CreateRecordItemsDesc()));
//mock the current data base context
var mockedDbContext = mocks.StrictMock<IDbContext>();
Expect.Call(mockedDbContext.CommitChanges);
SetupResult.For(mockedRepository.DbContext).Return(mockedDbContext);
mocks.Replay(mockedRepository);
return mockedRepository;
}
As you can see this method mocks the repository with specification for method calls return values and reveal the mocked repository to the outer world.
Now lets used this mocked repository to mock the service layer
public static IInventoryService CreateMockClipboardService()
{
var serviceMock =new InventoryService(InventoryRepositoryMock.CreateMockInventoryRepository());
return serviceMock;
}
Here the dependency injection is used with Windsor Castle to inject the service with the mocked repository layer.
Now to write a sample test using NUnit all you have to do is
[TestFixture]
public class ServiceTest
{
private IInventoryService _service;
[SetUp]
public void SetUp()
{
_service = new InventoryService(InventoryRepositoryMock.CreateMockInventoryRepository());
}
[Test]
public void GetWithoutSortTest()
{
var idList = new List<int> {1, 2, 3, 4};
const int truncLength = 100;
var itemsReturned = _service.Get(idList, truncLength);
var expectedItemType = typeof (ServiceItem);
const string expectedAuthor = "Dhanushka";
const string expectedTitle = "sample title";
Assert.That(itemsReturned.Count, Is.GreaterThan(0),"no items returned");
Assert.That(itemsReturned[0], Is.TypeOf(expectedItemType),"incorrect return type");
Assert.That(itemsReturned[0].Author,Is.EqualTo(expectedAuthor),"unexpected author returned");
Assert.That(itemsReturned[0].Title,Is.EqualTo(expectedTitle),"unexpected title returned");
}
}
Now we have a simple unit test in our hand that uses a mocked service and repository layer
Most of us use some sort of a mocking library when doing unit tests in order to mock our service and repository layers right!
Well I am a big fan of Rhino Mock and I have used it in almost all of my testing projects.
But one thing that remains to be a pain in the ass is creating test objects to be returned when the mocked repository is called. Well not any more!!!
Using NBulder we can avoid hard coding test objects for mocking purposes.
Here are some sample codes to get you started with
Let's say you want to mock a a repository call that returns an item, just write
var item = Builder<RepositoyType>
//set the id property of each items (Nhibernate is used with Windsor Castle in this occasion)
EntityIdSetter.SetIdOf(item, 1);
To mock a method call that returns a list of record objects.
var items = Builder<RepositoyType>
for (int i = 1; i < items.Count + 1; i++)
{
EntityIdSetter.SetIdOf(items[i - 1], i);
}
As you can see from the above example we can even set specific values for the attributes for returned objects as well.
And there are many more helper functions you can use to accomplish almost all the test object creation needs you hope for.
Happy Coding!!
I came across an interesting issue today. I used the ResetPassword method provided by C# membership class to reset the password of a user and found out that although it allows us to define the password strength using a regular expression in the web.config file, it does not guarantee that the generated password adheres to the rules defined by the expression. Microsoft says and I quote
"The random password created by the ResetPassword method is not guaranteed to pass the regular expression in the PasswordStrengthRegularExpression property. However, the random password will meet the criteria established by the MinRequiredPasswordLength and MinRequiredNonAlphanumericCharacters properties."
So to tackle the issue, I used the Regex class. The revised code is shown below
var isNotMatch = true;
while(isNotMatch)
{
resetpwd = manager.ResetPassword();
var matcher=new Regex(Membership.PasswordStrengthRegularExpression);
isNotMatch = !(matcher.IsMatch(resetpwd));
}
This code snippet will force the ResetPassword to fire until a password adhering to the rules defined by the regular expression is generated.