Yesterday I came across an interesting situation. There are some times when you need to break a string in to two parts depending on their character type.
Lets say you have a string like "Welfare Fund - 1000.00" and you need to break the string part and the number part in to two different strings. Well the first thing that comes to mind is iterate the string till you get a number and break it to apart upon hitting on a number type character, right!!
Well then what about a string like "Welfare Fund : 2009 - 3000.00"
Here as you can see, there are numbers in the string part as well.
The solution is simple, all you have to do is iterate through the string by starting from its end, till you reach a blank space. Here is the a method to accomplish that task
public static List<string> BreakString(string stringToBreak)
{
var charArr = stringToBreak.ToCharArray();
var stringAppender = new StringBuilder();
var intAppender = new StringBuilder();
var isSeparated = false;
for (int i = charArr.Length - 1; i > -1; i--)
{
if (isSeparated)
stringAppender.Append(charArr[i]);
else
{
isSeparated = charArr[i] == ' ';
if (!isSeparated)
intAppender.Append(charArr[i]);
}
}
var returnValues = new List<string> { ReverseString(stringAppender.ToString()), ReverseString(intAppender.ToString()) };
return returnValues;
}
public static string ReverseString(string s)
{
var arr = s.ToCharArray();
Array.Reverse(arr);
return new string(arr);
}
You can call it as follows
var input = "Welfare Fund - 1000.00";
var result = BreakString(input);
Welcome
"Happy are those who dream dreams and are ready to pay the price to make them come true"
About Me
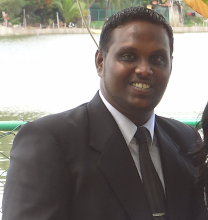
My Home @ google Map
- Dhanushka Athukorala
- Colombo, Western Province, Sri Lanka
- I'm a Microsoft Certified Professional (MCP-ASP.net) who is currently working as a Software Engineer @ Aeturnum. I have experience in windows, web, mobile application development using .net framework 2.0, 3.5, 4.0 and .net compact framework 2.0 and 3.5 and i have also carried out development projects in C++, C, VB.net, Java, PHP, Pl/SQL, SQL, XNA, AJAX, Jquery etc...
Followers
Posted by
Dhanushka Athukorala
Friday, December 24, 2010
Subscribe to:
Post Comments (Atom)